Case Study
MoviePool Full-Stack project
How I developed a responsive single-page application (SPA), a RESTful API, and a database, that provides movie lovers with information about movies and lets them manage their favorite movies and their user profile.
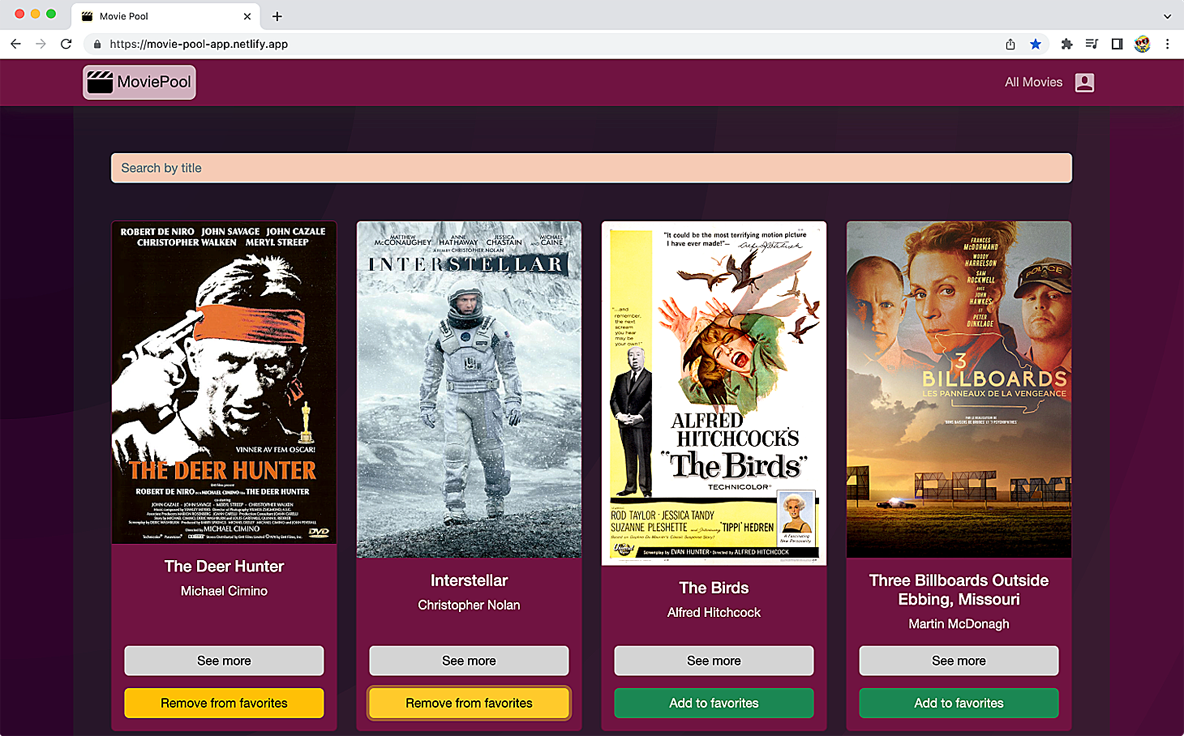
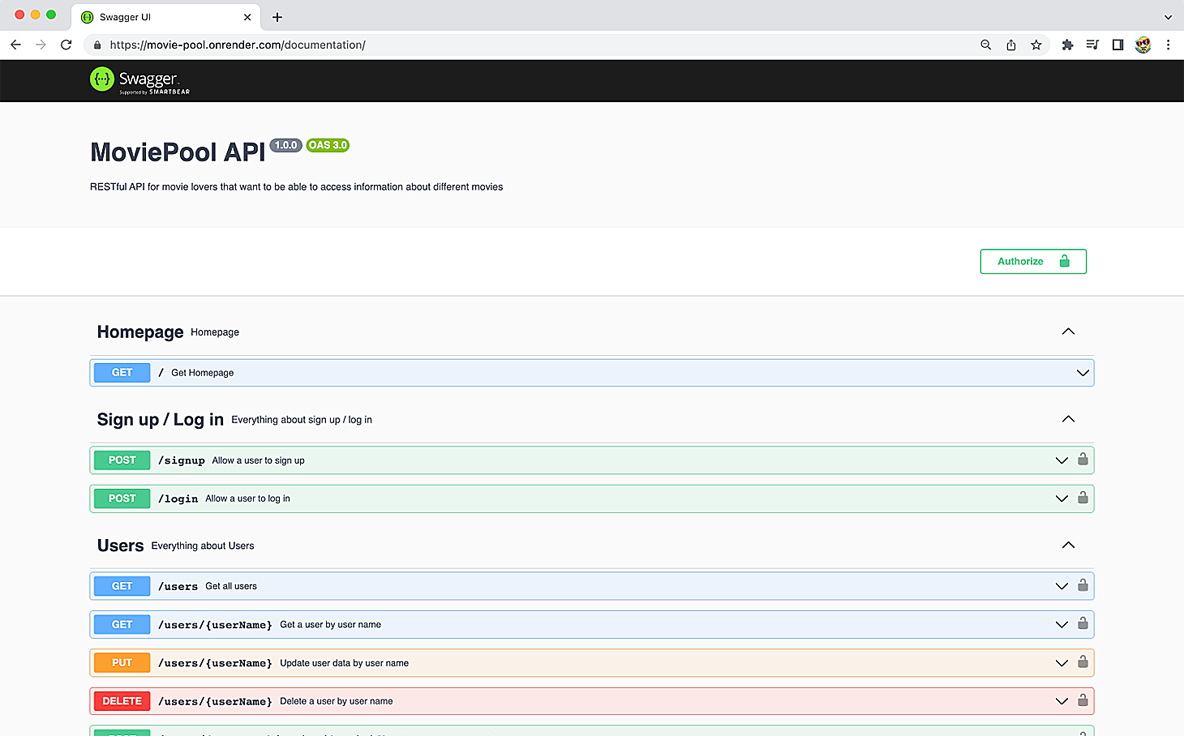
Overview
The MoviePool Full-Stack project consists of:
- a MoviePool RESTful API
- a NoSQL database, where data about movies and users is stored
- a MoviePool single-page application
Once a user has signed up, they can log in and are provided with access to a list of movies. They are able to browse through the list of movies, movie details like movie genres or movie directors, and can search a movie by movie title.
With sign up, a user automatically creates their user account where they then can update their personal information, manage a list of their favorite movies, and search for movies by movie title.
Context
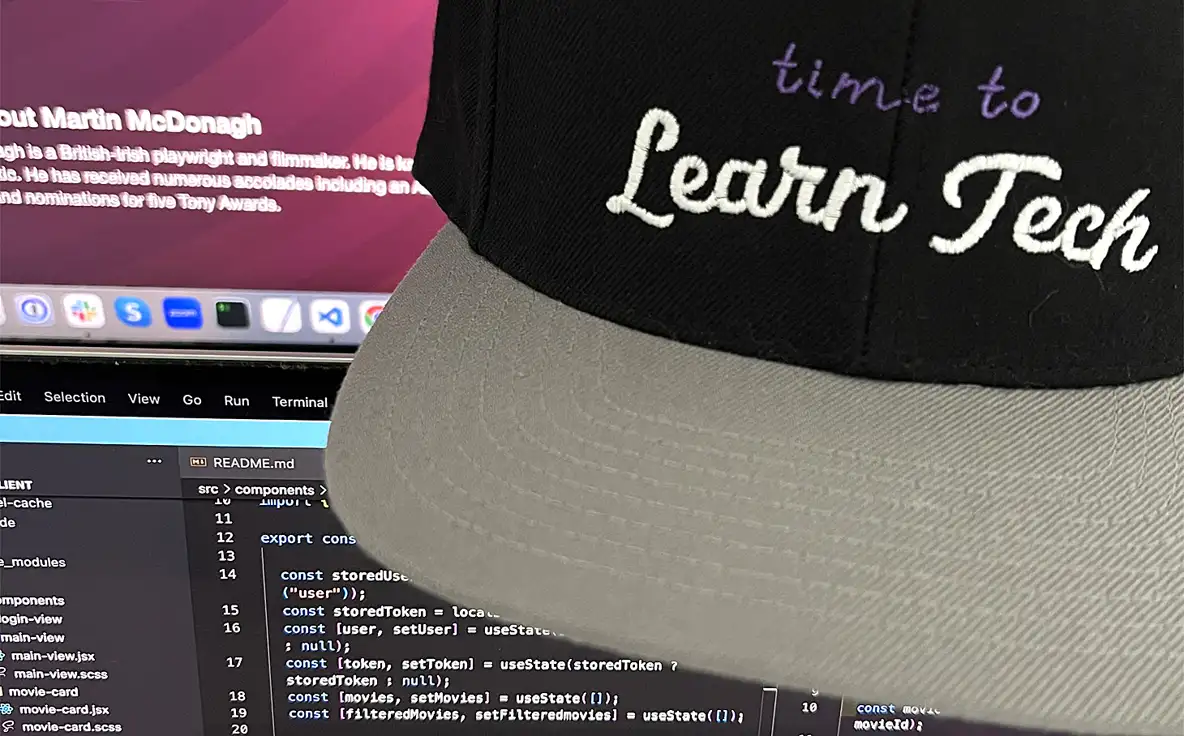
The MoviePool Full-Stack project is a project I built during my Full-Stack Web Development Program at CareerFoundry to pass Achievement 2 and 3.
Achievement 2 consisted of 10 exercises. Achievement 3 consisted of 9 exercises. Working from task to task led to the completion of the MoviePool Full-Stack project and demonstrates my mastery of Full-Stack JavaScript development, in this special case in the MERN stack.
Purpose & Objective
The goal of the MoviePool Full-Stack project was to build the server-side as a RESTful API, the client-side as a responsive SPA, and the NoSQL database from scratch, to provide users with access to a movie database and enable them to perform certain actions.
Styling was important but not the focus, as no Designers were involved.
Duration
The given timeline was 8 weeks to complete MoviePool RESTful API, MoviePool SPA and NoSQL database.
It took me 7 weeks to finish the MoviePool Full-Stack project and to pass the 19 exercises, I finished 1 week early.
Credits
A big thank you goes out to my tutor Blaise Bakundukize and to my mentor Stephen Barungi. Without their guidance and advice this task wouldn't have been as fun as it was.
Approach - MoviePool RESTful API
For the server-side, I developed a RESTful API that consists of 13 mandatory endpoints with a "code first" approach (first endpoints, then database), to receive data from and store data in a NoSQL database.
I use CRUD operations, Node.js as the JavaScript runtime environment because of its ability to run JavaScript on the server-side, Express.js as the web application framework as it already comes with functionalities I could use to create the API, and Swagger for API documentation.
The MoviePool RESTful API interacts with a non-relational database, also created by me.
Database
With MongoDB I developed a non-relational database that consists of two collections: users and movies.
I use Mongoose to create the business logic layer/models, and to connect to the database.
Authentication & Authorization
Authentication and authorization was implemented by me in the form of basic HTTP authentication, JWT, and Bcrypt for password hashing.
Once a user has signed up, they need to log in with username and password which will return a JWT (JSON Web Token), to ensure that only verified and authorized users can access the database and their information.
Testing & Documentation
I tested all endpoints in Postman, first locally, then live.
To document the API, I first used Postman, later changed to Swagger documentation (swagger-ui-express, yaml).
Hosting
The MoviePool RESTful API is hosted on Render.
The non-relational database is hosted on MongoDB Atlas.
Approach - MoviePool SPA
For the client-side, I developed the user interface as a responsive single-page application (SPA) in React.js, included React-Bootstrap components, with build tool being parcel.js, to enable a user to make requests to and receive responses from the MoviePool RESTful API.
Build Workflow
I use parcel.js as the build tool to automatically compile all of my files.
Components, Views & Design
Within React.js, I use React-Bootstrap components and Bootstrap, as this brings modular HTML set-up, styling and functionality out of the box. Additionally, I include my own styling.
I developed different views and use state routing to navigate between these views.
These views include:
- a sign up view - where a user can register via username, email, password, date of birth
- a login view - where a registered user can log in with their username and password
- a main view - that shows a list of all movies currently present in the database (as cards) and allows a user to add/remove a movie to/of their favorite movies list
- a single movie view - that shows information about a single movie and allows a user to add/remove a movie to/of their favorite movies list
- a profile view - where a user can update their user information, manage their list of favorite movies and delete their account
I also implemented a simple search in the main view, to let users search for a movie by title.
Hosting
The MoviePool SPA is hosted on netlify.
Retrospective
What went well
I loved building the whole MoviePool Full-Stack project! The development had me have a lot of aha moments I thoroughly enjoyed as they made me realize how satisfying (and fun!) it is to solve issues while being stuck and on the verge of giving up (meaning, reaching out to a senior developer), and then - BAM! - solving them myself.
Becoming aware that I am able to research myself silly to solve a problem was quite a confidence boost.
Challenges & Solutions
Complexity
Developing three products in parallel, first with static data, then hosted data, and to understand how a first SQL then NoSQL database, RESTful API, and SPA come together, interact and create requested results, was challenging.
Figuring out in what order to develop to get something successfully up and running (first locally, then hosted) was fun but also frustrating at times.
Solution: Breaking tasks down into small, manageable bits, succeed in solving them, understand more and more, bit by bit.
Errors & Bugs
To find out where errors and bugs were rooted was challenging, at the same time really fun!
I never knew that I would enjoy figuring out where errors or bugs come from so much, being it the database with a corrupted JSON object, the JavaScript or React.js syntaxes, a slow hosting provider, the build process, a slow database response... and then successfully solving them!
Solution: Monitoring ALL platforms, thoroughly reading ALL kinds of error messages, being it in the terminal, the browser developer console or on the page, researching, then solving issues, bit by bit.
Database Content
At the beginning the importance of a database with decent content was not clear to me.
Needed data I simply copy and pasted, partly from IMDB, partly from Wikipedia, to get those JSON objects filled up quickly, this made for a lack of good data quality as I rushed through this.
An example: at some point I had empty images (movie posters) on the page because I had no images in my JSON database. I simply forgot to research websites with movie posters to link to, and add those links to my JSON objects. Besides a first panic attack, I then researched and added the links to my database movie objects at MongoDB Atlas directly.
Solution: Taking an extra minute to go through requirements and to make sure to understand the role of every single detail. It might turn out to be important to put more effort in the beginning to not have extra work in the middle or at the end.
Authentication & Authorization
Understanding the different layers and order of processes to authenticate and authorize was tough.
I still need some clarification about what is sent when to receive what kind of result, with which kind of authentication/authorization, choosing from a multitude of middleware and packages thrown at the API.
Solution: I've already built a additional mini MERN project, following my "break it down into smaller bits to better understand" motto. This only serves as a start to clarify questions I have regarding authentication and authorization.
React-Bootstrap Components & Bootstrap
It was challenging to understand in a very short amount of time what React-Bootstrap components and Bootstrap bring out of the box (HTML/JSX, styling, functionality), and what to keep as for the MoviePool SPA, it was expected to use specific React-Bootstrap components within a specific React set-up.
The time-based decision to not go into deep detail on how to best apply custom styles to mandatory React-Bootstrap components or even to look into Bootstrap theming, was challenging.
This conscious decision led to overwriting present Bootstrap styles which might not even be needed, and on top of that does not really resulted in the best visual experience, is inefficient and makes the code messy.
Solution: Working on React-Bootstrap, Bootstrap projects in the future, I would make and take the time needed to go through React-Bootstrap/Bootstrap documentation.
Final Thoughts & Outlook
I'm proud of what I've developed with MoviePool RESTful API, MoviePool single-page application and NoSQL database, in a short period of time. Getting my head around this and (free) hosting options was fun and exciting.
I've already decided that I do not want to refactor these single projects as this would take too much time I'd rather spend in building new projects that are based on what I've learned, for example the mini MERN project I've mentioned earlier.
If I would consider refactoring, I would definitely refactor the MoviePool SPA. Its styling only contains the bare minimum and accessibility was not seriously taken into account.
Looking forward to my next endeavors in the MERN stack!
Links
MoviePool API demo on render MoviePool API code on GitHub MoviePool App demo on netlify MoviePool App code on GitHubPublished: July 2023